This indicator calculates the lot size based on the balance percent risked per trade. It takes into account the commission paid to the broker.
Input Parameters
- StopLossPips: the stoploss in pips of the trade.
- RiskPerTrade: the balance percent risked. (0.01 means 1%, 0.25 means 25% and so on…)
- RoundTurnCommissionPerLotPips: the commission charged by the broker expressed in pips.
- TextPosition: indicates the vertical line at which the results are printed. It is used to prevent text overlapping when multiple indicators print at the same line.
Screenshots
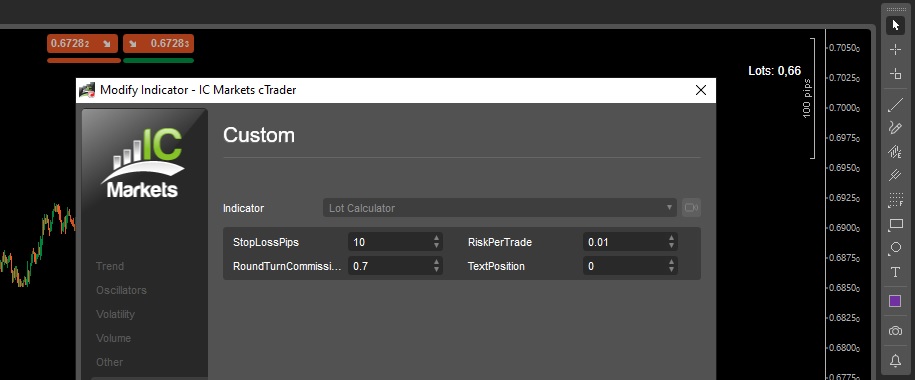
Code
using System; using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; using cAlgo.Indicators; namespace cAlgo { [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class LotCalculator : Indicator { [Parameter(DefaultValue = 10, MinValue = 0)] public double StopLossPips { get; set; } [Parameter(DefaultValue = 0.01, MinValue = 0, MaxValue = 1)] public double RiskPerTrade { get; set; } //RoundTurnCommissionPerLot [Parameter(DefaultValue = 0.7, MinValue = 0)] public double RoundTurnCommissionPerLotPips { get; set; } [Parameter(DefaultValue = 0, MinValue = 0)] public int TextPosition { get; set; } private string line; protected override void Initialize() { line = ""; for (int i = 0; i < TextPosition; i++) line += "\n"; } public override void Calculate(int index) { double lots = (Account.Balance * RiskPerTrade) / (StopLossPips + RoundTurnCommissionPerLotPips) / Symbol.PipValue / 100000; Chart.DrawStaticText("lots", line + "Lots: " + lots.ToString("0.00"), VerticalAlignment.Top, HorizontalAlignment.Right, Color.White); } } }